Convert Python Code Into a Pip Package
2023-02-28
843 words
4 mins read
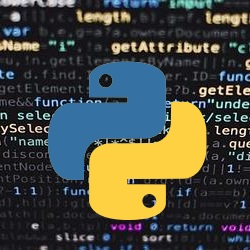
This article describes how to convert your Python code into a Pip package.
Initial Steps
Arrange your code into the proper file hierarchy Add your init.py files Add a license and a README.md if not already done
Note: At any time, you are welcome to refer to my example Github Repo to see the file structure
File Hierarchy and init files
/demopackage
__init__.py
demopackage.py
/demosubpackage
__init__.py
demosubpackage.py
/tests
test_package.py
LICENSE
README.md
setup.py
When you want to create a python module, such as when you import locally or you are building a python library, it is best practice to add it in a folder with an init.py file that contains:
name = 'demopackage'
This file allows Python to easily find modules and sub-modules so that they can be imported.
Note: The name of your main folder/module will be the name of your Python library, so pick something you like!
Adding a License
“Any source code you distribute must have a license attached to it, even if you intend for it to be fully open source. If there is no license attached to your code technically it isn’t legal for anyone to use it since all creative work has an exclusive copyright attached to it by default.
This page from the Hitchhiker’s Guide to Python does a good job introducing you to the available licenses and links to a couple of tools to help you choose.
Most python libraries use MIT or BSD licenses, which are very permissive open-source licenses. Being more permissive makes it easier for a wide variety of potential users to use, especially users in the industry. Keep in mind that GPL licenses are “viral” meaning that if you incorporate source code that uses a GPL license, your code must also have the more restrictive GPL license.
The argument I hear most often from fellow researchers for using a more restrictive license, like the GPLv3, is that they ensure your code always remains free and is never incorporated into a private code base.” - Will Norris
Publish Your Package to PyPI
PyPI refers to the [Python Package Index](https://pypi.org/). This is the repository for python packages that are installable via pip. After following these four simple steps, you will have uploaded your very own Python library you can use, distribute, or share with anyone!
- Sign-up for a PyPI account and verify your email address
- Create your setup.py file
- Build distribution archive files
- Upload!
Create your setup.py file Your setup.py file is your build script for setuptools, which is the library that packages your code. Your setup.py file is essentially what holds the most basic information about your package and where pip can find the source code. Every time you update your package, you will change the version number in your setup.py before uploading it to PyPI.
Here is an example setup.py file:
import setuptools
with open("README.md", "r") as fh:
long_description = fh.read()
setuptools.setup(
name="demopackage",
version="1.0.0",
author="demo_author",
author_email="[email protected]",
description="A small demo package",
long_description=long_description,
long_description_content_type="text/markdown",
url="https://github.com/username/demopackage",
packages=setuptools.find_packages(),
classifiers=(
"Programming Language :: Python :: 3",
"License :: OSI Approved :: MIT License",
"Operating System :: OS Independent",
),
)
Note: For a full list of classifiers you can use in your setup.py file, check out this link from PyPI’s Docs.
Create your Distribution Archive Files
Before you start, run the following command to update the necessary packages:
python -m pip install --user --upgrade setuptools wheel
Next, make sure you are in the same directory as the setup.py file (main project directory), and then run:
python setup.py sdist bdist_wheel
This will create a ‘dist/’ folder in your main directory and the compressed files for your package!
Upload your package archives to PyPI
Verify that you have twine installed:
python -m pip install --user --upgrade twine
Twine helps manages the file upload to PyPi. Next, run this command:
twine upload dist/*
If you don’t pass a location for twine to upload your distribution archives, it will default to the PyPI legacy servers, which is what we want!
You will be asked for your PyPI login credentials, and then the upload will begin. Now you can log in to your PyPI account and you will see your package. PyPI also displays your README on the package’s main page, so utilize that space to give useful information about your library.
Test Your New Package
Wait 5–10 mins. for your uploaded package to be added by pip. Sometimes it takes a few minutes, other times you can install it right away!
pip install demopackage
Maintaining a pip Package
Anytime you want to make improvements to your code, you will have to re-upload new distribution files to PyPI following the same procedure.
Key Steps:
- Changing the version number in your setup.py file makes versioning much easier.
- Remove old distribution version files
- In your package’s main folder directory run:
to create new distribution package archives.pip install demopackage python setup.py sdist bdist_wheel
- Upload the new version to PyPI:
twine upload dist/*
Wrapping Up
Congratulations! You now have your very own Python library that anyone can install and make use of in their workflows.
Authored By Is-Rael Landes
Is-Rael Landes, a good man living on the earth, loving making website, teaching others and coding.